Session Timeout Error in Ajax Applications
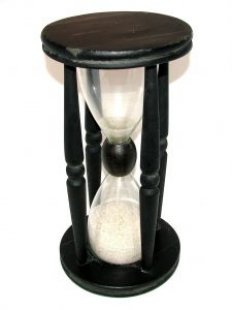
I recently discovered a major issue in many of my(and others) Ajax apps - not handling Session timeout properly. Lets say that you are using PHP as the backend. It has a 20 minute time limit for sessions(by default). If the user does not make any requests in that time, the session will get timed out. In normal, web 1.0, non-ajax applications, this is not a problem. If the session variable is not set, the code will do a redirect to the login page. But in Ajax applications, this becomes a problem.
This problem affects only the applications that uses logins and user authentication within their Ajax apps. Also, if you are using polling in any page, that page should not be affected. each polling request will be considered as an interaction.
Find if your App has this error
Login into your application - don't use remember me if your app has that feature. Next, go to a page where you will be able to send an Ajax request. Now wait for about half an hour without interacting with the site. Don't click on any links, don't go to other pages of the site - don't do anything. After half an hour, make the Ajax request. If the page acts weirdly(JavaScript Error, invalid return data, etc), your app has the problem.
How it should be...
Ideally, this is what the code should do in such cases.
- An Ajax request is made
- Server Code checks if the session variable is set
- If the session variable is set, send the data.
- If the session does not exist, send an error message to the client side
- JavaScript should intercept this error message
- Do a JavaScript redirect to the login page if this error exists.
The problem is that this should be done for every single request. If you already have an application that has this issue, there is a considerable amount of coding ahead of you.
Solution
success/error Design Pattern
I use a success/error design pattern for Ajax requests - each response is in JSON format and has a minimum of two elements in it - ie 'success' and 'error' - like this...
{
"success":"Task created successfully",
"error":false
}
The return handler function will be something like this...
function ajaxReturn(data) { //data is the 'eval'ed object of the responseText
if(data.error) {
//The 'showErrorMessage' handles the error.
//showErrorMessage() will return true for a critical error and false for non-critical error.
if(showErrorMessage(data.error)) return;
}
//Do whatever you want with 'data'
alert(data.success);
}
I then set up the 'showErrorMessage' function to handle the 'session timeout' error with a redirect.
function showErrorMessage(error_message) {
if(error_message == 'User not logged in' || error_message == 'SESSION_TIMEOUT') {
alert("Your session was timed out due to inactivity. Please Login to continue"); //Show an error message - or not - your choice.
document.location.href = '/login.php'; //...and redirect to login page.
return true;
} else if(error_message == 'another error') {
//Handle 'another error'
/* ... */
} else {
$("error-message").innerHTML = error_message; //Default error handling - just show the error message to the user.
}
return false;
}
When using AHAH
If you are using the AHAH method, it could get a bit more complicated. The best thing to do is to change to a JSON based approach. But if you cannot do that, the only method I can think of is return a login form as the Ajax response. This form will get inserted into the document. I will not recommend this method - the problems with this method is too numerous to list here.
Do you know any other method to solve this problem?
blog comments powered by Disqus