Changing Appearance of Elements using Style
Popups
Moving Stuff around
Moving Stuff around
With javascript, you can move stuff(pictures, text, tables, etc.) around the page. Not very useful - but very cool. If you master this technique, you can even make games with javascript - but don't try to make Max Payne III with it - I believe the title is copyrighted by its creators - they could sue.
Before starting, let me warn you that moving things have very little practical use in javascript. You could make games - but your games will be very limited if you chose javascript as your programming language. There are two things that are of practical advantage - the top
and left
property and 'setTimeout
' command. So pay special attention to them.
For moving an item, we use the 'top' and 'left' property of the item. First, let us display an image. The following image was made with the code...
<style>
DIV.movable { position:absolute; }
</style>
<div id="ufo" class="movable"><img src="images/ufo.gif" /></div>
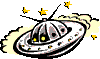
See the 'style' tags? Put that in the HEAD area of the document. This will cause the image to be positioned absolutely. This is very important if we want to move the image. When we move the image, we are actually giving it an alternate position. Try it with the code...
document.getElementById("ufo").style.top = '600px';
document.getElementById("ufo").style.left = '550px';
This code will move the image to position 600 pixels below the top of the document and 550 pixels from the left of the document. The below 'image' will illustrate how the image was displayed. Please note that the top will be counted from the top of the document - not from the top of the window. Even if you have scrolled below a lot, the top will be from the start of the document.
+------------------------+
| ^ |
| | |
| | |
| 600px |
| | |
| | |
| v |
|<--550px-->+-------+ |
| | Image | |
| +-------+ |
| |
+------------------------+
Why not try a little exercise here? Try to put the UFO picture inside the red box called image(above). You can use the textarea given above to do it - guess its top and left co-ordinates by using the trail and error method. If you fail to do it, highlight the below table(triple click the below box) to see the code that does it.
document.getElementById("ufo").style.top = '930px'; document.getElementById("ufo").style.left = '210px'; //Please note this will depand many factors - like your screen resolution - // so the image may not be positioned correctly |
Now we will try to move the image - in a way that we will see it moving. The above given method will just take a image and make it appear at a different location. In the next method it will move slowly to the next location. We will do this by moving the image to a different location by moving it a bit at a time. For example if we want to move the image to the position 300(top) and 300(left) from the position 5(top) and 5(left), we will move it to 10 pixels at a time till it reach the desired location - this will give the viewer an illusion of motion. Click here to see it in action.
A note about the below code - it does not work with the Test Box. But it is a vaild code. To experiment with it, please create a HTML file in your system and insert this code in the HTML file and then it will work properly.
<html>
<head>
<title>Image Mover</title>
<style>
DIV.movable { position:absolute; }
</style>
<script language="javascript">
var x = 5; //Starting Location - left
var y = 5; //Starting Location - top
var dest_x = 300; //Ending Location - left
var dest_y = 300; //Ending Location - top
var interval = 10; //Move 10px every initialization
function moveImage() {
//Keep on moving the image till the target is achieved
if(x<dest_x) x = x + interval;
if(y<dest_y) y = y + interval;
//Move the image to the new location
document.getElementById("ufo").style.top = y+'px';
document.getElementById("ufo").style.left = x+'px';
if ((x+interval < dest_x) && (y+interval < dest_y)) {
//Keep on calling this function every 100 microsecond
// till the target location is reached
window.setTimeout('moveImage()',100);
}
}
</script>
</head>
<body onload="moveImage()">
<div id="ufo" class="movable">
<img src="ufo.gif" alt="Please link to a vaild image." />
</div>
</body>
</html>
That will move the image smoothly to its new location. Feel free to experiment with the above code.
Moving is not limited to images - you can move text, tables or other HTML entities. Just put it in a DIV tag and move that tag. An example...
Why are research scientists now using lawyers instead of rats in laboratory experiments?
|
Why should all lawyers be 6 Feet under?
Because deep down, they're nice guys. Why is five lawyers in a buick going off a cliff a sad thing? A buick holds six. |
Now let us inter-change the two jokes - please note that this may NOT be accurate - as it will depend on your resolution.
document.getElementById("joke_1").style.left = '420px';
document.getElementById("joke_1").style.width = '300px';
document.getElementById("joke_2").style.left = '120px';
document.getElementById("joke_2").style.width = '290px';
The first line will take the first joke and put it in the position of the second joke(430 pixels). The third line will take the second joke and put it in the place of the first joke(130 px). I hope you remember the 'width' property from the last chapter.
blog comments powered by Disqus