Image Control
Now we move on to a more interesting use of javascript - manipulating images. First of all let us display an image.
<img src="images/bear.gif" alt="Picture of a Bear" />
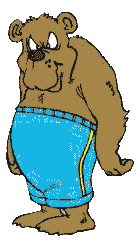
Wait! This is just plain HTML displaying of images. JavaScript was not used. So let us see a image that is shown using javascript...
<img name="img1">
<script>
document.images['img1'].src = "images/surgeon.gif"
</script>

In the above example, we are accessing the object called 'img1' and changing its 'src' or the source value. In effect, we are changing the picture. You can change other items like image height and width also.
document.images['img1'].src = "images/surgeon.gif"
document.images['img1'].width = 150
document.images['img1'].height = 200
Image also have other values like border, hspace, vspace etc. Now let us see how to create an Image object.
//Create the image object
var image_object = new Image();
image_object.src = "images/scientist.jpg"; //Preload the Images.
document.images['img1'].src = image_object.src; //Display the image
This will preload the image. That means that if the code is placed at the top of the file the response time will be decreased. With a little imagination you can do a lot with this little bit of code. Think of the possibilities - create an array of images and display it as animation. Or create a slide show with your images. Or you could create the most commenly seen trick with images - the roll over effect.
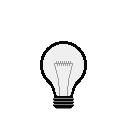
The above example is a mouse over effect. When you move your mouse over the given image, the image will change into some other image. Creating this effect is simple. First of all, we need the primary image.
<img name='roller' src="images/roll1.gif">
Now we add a function that will be called when the moves moves over the picture.
<img name='roller' src="images/roll1.gif" onMouseOver="changer()">
After that lets create the function 'changer()' that will do the actual changing of the picture.
<script language="javascript">
function changer() {
document.images['roller'].src = "images/roll2.gif"
}
</script>
<img name='roller' src="images/roll1.gif" onMouseOver="changer()">
Voila! Now we have a picture that will change when the mouse comes over it. Wait a minute - when the mouse moves away, the picture don't change back. We need to change that don't we?
<script language="javascript">
//Preload both the images
var images = new Array(2);
images[0] = new Image();
images[0].src = "images/roll1.gif"
images[1] = new Image();
images[1].src = "images/roll2.gif"
//Toggles the image
function changer() {
if(document.images['roller'].src == images[0].src) document.images['roller'].src = images[1].src
else document.images['roller'].src = images[0].src
}
</script>
<img name='roller' src="images/roll1.gif"
onMouseOver="changer()" onMouseOut="changer()">
Now we have a fully functional MouseOver effect. I have to admit that there is no need to use all this code to create such a simple effect. I just wanted to show you how to do it is done using the image constructor. Now let us see the easy way to do it.
<img name='roller' src="images/roll1.gif" onMouseOver="this.src='images/roll2.gif'" onMouseOut="this.src='images/roll1.gif'">
The problem with the above method is that the browser will not load the second image into the memory - making a pause between the mouseover event and the image chage. To avoid this, use the fully functional method - the former one.
blog comments powered by Disqus